Variables in Python: Python For Beginners
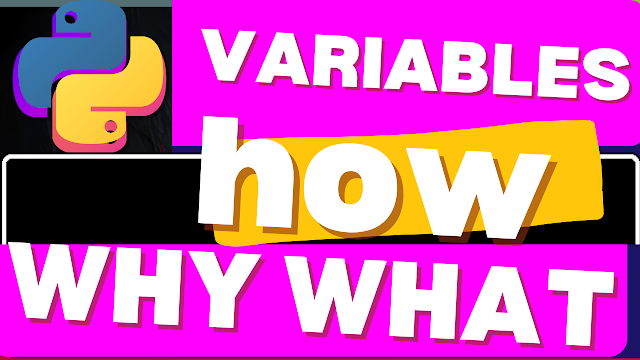
Tuples allow storage of multiple items using a single variable. Tuples are frequently used in Python for storing data and every programmer needs to know all about tuples in order to code efficiently in Python.
Tuples could be considered as a collection containing multiple data items that are unchangeable and ordered and also enclosed in round brackets. Tuples are infact inbuilt data structures that store different types of data in one variable. Tuples use round parentheses to store data.
Below, in the code, you can understand, how a tuple is created. The variable mytuple is a tuple containing multiple items, that are string items , namely, 'cat', 'dog' and 'rabbit'. We use the round brackets to enclose the three items as shown below and you can use the print function to get the resultant tuple containing these items.
mytuple = ("cat","dog","rabbit")
print(mytuple)
As mentioned above already, tuple items are unchangeable and ordered and they also permit duplicate values in the tuple.
In the code below, we use the value of boolean, True twice in the tuple , mytuple3.
The data types of items contained in a tuple could be string, booleans and integers. At any point of time, a single tuple could either store just strings or booleans or integers or a mix of strings, booleans and integers, as demonstrated below.
#tuples data types
mytuple1 = ("John","Tom","Tim")
mytuple2 = (2,7,4,5)
mytuple3 = (True,False,True)
print(mytuple3)
#mix data types
mytuple = ("xyz","Sara",False,70)
print(mytuple)
Tuples cannot be changed after it is created and we cannot add or remove items either to a tuple , after it is created. The order of the tuple items also cannot be changed after it is create.
In mytuple containing the three numbers, 1,4,7, if we try to assign a new value to the first number in this tuple , then, we will see an error message since we are not allowed to change items once a tuple has been made and item assignment is not permitted in the case of tuples. However, if the tuple is inside a list, then, the items can be changed as demonstrated below whereby, the num list contains a tuple with three tuple items namely (2,7),(1,4),(4,9) and we assign a new value for the tuple item with index value of 1, that is, the second tuple item within this list. The new value assigned to the item (1,4) is (5,7) and when we print this item, we can see that it prints out the new value that is assigned to it. Hence, we conclude that tuples can only be changed if it is contained inside a list as shown below.
#tuple is not changeable
mytuple = (1,4,7)mytuple[0] = 2print(mytuple)
#above code throws an error
#tuples inside list
num = [(2,7),(1,4),(4,9)]
print(num)
print(num[1])
num[1] = (5,7)
print(num[1])
We can determine the length of a tuple by using the len() function as shown below on the tuple, mytuple which has 6 string items. When we use the len() function here, and print out the result, we get the result as 6, since there are 6 items in the tuple and the len() function will determine the length of the tuple or in other words, the number of items in a tuple.
mytuple = ("rose","ann","rose","tom","ann","rose") print(mytuple)
print(len(mytuple))
We can create new tuples by using the tuple() constructor.
In the below example we create a new tuple by the name of mynewtuple using the tuple() constructor and the items that we include in the tuple that we are creating, but, we need to use the double round brackets since one set of round brackets is for the constructor and the other set of round brackets is for enclosing the tuple items, in this case, they are the string items.
The resultant tuple namely, mynewtuple , as shown in the code below, would be showing up in the result as ("Tiger", "Lion", "Mouse", "Rabbit")
Tuples accept duplicate values for item storage, be it in strings, or booleans or integers. You can check it out by observing the tuple below called mytuple with duplicate values for the string item, rose and ann. Please refer to the code below.
#tuple constructor
mynewtuple = tuple( ( "Tiger", "Lion", "Mouse", "Rabbit" ) ) print(mynewtuple)
#allows duplicates mytuple = ("rose","ann","rose","tom","ann","rose")
print(mytuple)
We can create a tuple with just one item, but, for doing this, we need to add a comma after the single item even if there are no more items after the first item. Please refer to the example code below to check how to create a tuple with just one item , that is, a string item, "Sarah".
#tuple with one item
mytuple = ("Sarah",)
print(mytuple)
I have explained all about tuples in the above paragraphs with specific examples, however, just for the sake of convenience, I have also put together, all the code examples in a code editor file as shown below, for your reference to enable you to practise lot of times to get a hang of this topic.
mytuple = ("cat","dog","rabbit")
print(mytuple)
#allows duplicates
mytuple = ("rose","ann","rose","tom","ann","rose")
print(mytuple)
print(len(mytuple))
#tuples data types
mytuple1 = ("John","Tom","Tim")
mytuple2 = (2,7,4,5)
mytuple3 = (True,False,True)
print(mytuple3)
#mix data types
mytuple = ("xyz","Sara",False,70)
print(mytuple)
#tuples inside list
num = [(2,7),(1,4),(4,9)]
print(num)
print(num[1])
num[1] = (5,7)
print(num[1])
#tuple with one item
mytuple = ("Sarah",)
print(mytuple)
#tuples store items
#with strings
tuple1 = ("jan","feb","mar")
print(tuple1)
#with numbers
tuple2 = (1,2,3,4)
print(tuple2)
#with booleans
tuple3 = (True,False,True)
print(tuple3)
#with mixed type data
tuple4 = (1,True,"jan",4)
print(tuple4)
#nested tuple
tuple5 = ("rat",[1,2,3],(4,7,6))
print(tuple5)
#tuple inside list
tuple6 = [(1,7),(4,5),(7,7)]
print(tuple6)
#tuple can store duplicate data
tuple7 = ("sara","march","sara","jan","march")
print(tuple7)
#with one item
tuple8 = ("jan",)
print(tuple8)
#check if tuple
print(type(tuple8))
#if no comma its string
tuple9 = ("feb")
print(tuple9)
print(type(tuple9))
tuple10 = (1,5,7,5)
#chk length of tuple
print(len(tuple10))
#tuple not mutable
#mytuple = (1,4,7)
#mytuple[0] = 2
#print(mytuple)
#mutable if tuple inside list
tuple11 = [(3,5),(1,2),(4,7)]
tuple11[0] = (7,9)
print(tuple11)
#tuples store items#with strings
tuple1 = ("jan","feb","mar")
print(tuple1)
#with numbers
tuple2 = (1,2,3,4)
print(tuple2)
#with booleans
tuple3 = (True,False,True)
print(tuple3)
#with mixed type data
tuple4 = (1,True,"jan",4)
print(tuple4)
#nested tuple
tuple5 = ("rat",[1,2,3],(4,7,6))
print(tuple5)
#tuple inside list
tuple6 = [(1,7),(4,5),(7,7)]
print(tuple6)
#tuple can store duplicate data
tuple7 = ("sara","march","sara","jan","march")
print(tuple7)
#with one item
tuple8 = ("jan",)
print(tuple8)
#check if tuple
print(type(tuple8))
#if no comma its string
tuple9 = ("feb")
print(tuple9)
print(type(tuple9))
tuple10 = (1,5,7,5)
#chk length of tuple
print(len(tuple10))
#tuple not mutable
#mytuple = (1,4,7)
#mytuple[0] = 2
#print(mytuple)
#mutable if tuple inside list
tuple11 = [(3,5),(1,2),(4,7)]
tuple11[0] = (7,9)
print(tuple11)
To conclude, Tuple is a collection of data items that are immutable and ordered.
Tuples also allows duplicate values inside the variable container as shown in the various examples above.
We can determine the length of the tuple by using the len() method as mentioned in the example codes . Tuples can store string items, numbers and also booleans. In fact, tuples can also store a mix of different data types such as a mix of string, number and boolean.
Tuples can also be stored inside lists as mentioned earlier . Tuple with one item needs to be shown with a comma after the item contained or else it will throw an error. Tuples are not mutable, that is, you cannot change the value of the items stored inside a tuple. However, if the tuple is stored inside a list as shown in the num tuple below, then, you can access and change the value of the item inside the tuple, due to the mutable nature of the lists.
Just as in the case of lists, tuples can also access the items inside it with the use of index keys however, it cannot modify the values of items inside the tuples unless the tuple itself is contained inside a list as shown in the code above.
Related topic you may want to read:
Comments
Post a Comment