Variables in Python: Python For Beginners
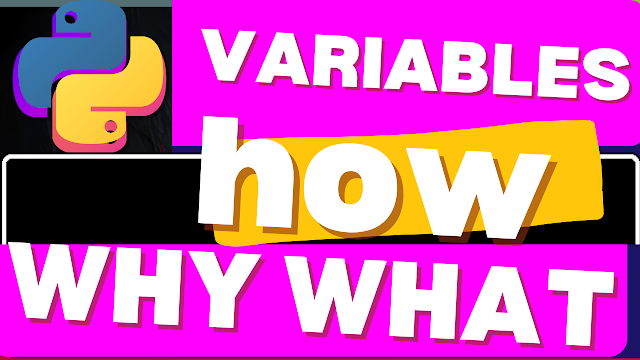
Build A Random Quote Machine in React
This ReactJS Tutorial will teach you how to create a random quote generator using React hooks. This is a challenge for testing your frontend libraries in the freeCodeCamp frontend libraries certification projects and in this challenge, I will walk you through the steps so that you can pass all the ten tests in this challenge.
This React Project is part of the freeCodeCamp frontend Libraries Certification Challenges and here, I am going to walk you through the challenge and also break down the challenge into smaller, more understandable steps, to demonstrate how to build this App in a simple way.
The Random Quote Machine App is a very unique app that will allow the users to interact with the app , whereby by clicking on a button, you will be able to see random new quotes with their authors' names and you will also be able to share these quotes on Twitter.
Code editors required for this challenge :
We will be coding in HTML, CSS and JavaScript files. For this project, I have coded on two cloud editors, namely, CodePen and also Jsitor. You could sign up for a CodePen account if you don't have one or you could also sign up for a Jsitor account.
Before we start coding a project , it is essential to understand the project in a no-code or pseudocode way, that is, make a reality check and rough layout of the project and consider what it would like as a finished product and what are the main ingredients that are part of the output in order to tackle the whole process smoothly without any hickups.
The Pseudocode for understanding the project challenge:
We consider the layout of the App that we are going to build, that is, the Random Quote Machine , with the help of the diagram above. In the above picture, I have taken a screenshot of the actual image of the app output) , we notice the following :
There is a main container with another box inside this container and this box termed, the QuoteBox container with a blue background in the main container. The QuoteBox has following :
There are some lines of quotes and below the quote, we see the author's name.
There is a button called 'New Quote' button and another button beside it called the 'Tweet' button.
The QuoteBox container with the above items also has a colored background. Current color of the QuoteBox background is black, but, it is supposed to keep changing colors every time the quote keeps changing or rather every time, a new random quote appears on the screen when the user clicks on the New Quote button. The purpose of the Tweet button beside the New Quote button, is to share the new quotes that appears here, on the Twitter landing page.
Code for the HTML file:
In the HTML file, we write the below code. This means the main component is the App Component and the rest of the components and elements we will be building inside this main app. The app has a container with a div id of "app". Hence, this explains the code entered below.
The code in HTML file:
<div id="app"></div>
const quoteData = [ { text: "The elevator to success is out of order. You’ll have to use the stairs, one step at a time.", author:"Joe Girard" }, { text: "People often say that motivation doesn’t last. Well, neither does bathing – that’s why we recommend it daily.", author:"Zig Ziglar" }, { text: "I always wanted to be somebody, but now I realise I should have been more specific.", author:"Lily Tomlin" }, { text: "I am so clever that sometimes I don’t understand a single word of what I am saying", author:"Oscar Wilde" }, { text: "People say nothing is impossible, but I do nothing every day.", author:"Winne the Pooh" }, { text: "Life is like a sewer… what you get out of it depends on what you put into it.", author:"Tom Lehrer" }, { text: "You can’t have everything. Where would you put it?", author:"Steven Wright" }, { text: "Work until your bank account looks like a phone number.", author:"Unknown" }, { text: "Change is not a four letter word… but often your reaction to it is!", author:"Jeffrey Gitomer" }, { text: "People often say that motivation doesn’t last. Well, neither does bathing – that’s why we recommend it daily.", author:"Zig Ziglar" }, { text: "I always wanted to be somebody, but now I realise I should have been more specific.", author:"Lily Tomlin" }, { text: "I am so clever that sometimes I don’t understand a single word of what I am saying", author:"Oscar Wilde" }, { text: "People say nothing is impossible, but I do nothing every day.", author:"Winne the Pooh" }, { text: "Life is like a sewer… what you get out of it depends on what you put into it.", author:"Tom Lehrer" }, { text: "You can’t have everything. Where would you put it?", author:"Steven Wright" }, { text: "Work until your bank account looks like a phone number.", author:"Unknown" }, { text: "Change is not a four letter word… but often your reaction to it is!", author:"Jeffrey Gitomer" }, { text: "If you think you are too small to make a difference, try sleeping with a mosquito.", author:"Dalai Lama" }, { text: "People often say that motivation doesn’t last. Well, neither does bathing – that’s why we recommend it daily.", author:"Zig Ziglar" }, { text: "I always wanted to be somebody, but now I realise I should have been more specific.", author:"Lily Tomlin" }, { text: "I am so clever that sometimes I don’t understand a single word of what I am saying", author:"Oscar Wilde" }, { text: "People say nothing is impossible, but I do nothing every day.", author:"Winne the Pooh" }, { text: "Life is like a sewer… what you get out of it depends on what you put into it.", author:"Tom Lehrer" }, { text: "You can’t have everything. Where would you put it?", author:"Steven Wright" }, { text: "Work until your bank account looks like a phone number.", author:"Unknown" }, { text: "Change is not a four letter word… but often your reaction to it is!", author:"Jeffrey Gitomer" }, { text: "If you think you are too small to make a difference, try sleeping with a mosquito.", author:"Dalai Lama" }, { text: "People often say that motivation doesn’t last. Well, neither does bathing – that’s why we recommend it daily.", author:"Zig Ziglar" }, { text: "I always wanted to be somebody, but now I realise I should have been more specific.", author:"Lily Tomlin" }, { text: "I am so clever that sometimes I don’t understand a single word of what I am saying", author:"Oscar Wilde" }, { text: "People say nothing is impossible, but I do nothing every day.", author:"Winne the Pooh" }, { text: "Life is like a sewer… what you get out of it depends on what you put into it.", author:"Tom Lehrer" }, { text: "You can’t have everything. Where would you put it?", author:"Steven Wright" }, { text: "Work until your bank account looks like a phone number.", author:"Unknown" }, { text: "Change is not a four letter word… but often your reaction to it is!", author:"Jeffrey Gitomer" }];;
const backgroundColors = ["#F0F8FF","#FAEBD7","#00FFFF","#7FFFD4","#FFE4C4","#000000","#FFEBCD","#0000FF","#8A2BE2","#A52A2A","#DEB887","#5F9EA0","#7FFF00","#D2691E","#FF7F50","#6495ED","#DC143C","#00FFFF","#00008B","#008B8B","#8B008B","#556B2F","#9932CC","#8B0000","#483D8B","#00CED1","#9400D3","#FF1493","#1E90FF","#FF00FF","#FF69B4","#00FF00","#0000CD","#4169E1","#2E8B57","#008080","#FFFF00","#FF6347","#D8BFD8","#9ACD32","#6A5ACD","#FA8072","#DDA0DD","#800080","#CD853F","#AFEEEE","#FF4500","#C71585","#191970","#BA55D3","#20B2AA"];
/*const backgroundColors = ["blue","red","pink","brown","yellow","green", "magenta"];*/
const QuoteBox = ({ quote, handleNewQuote }) => ( <div id="quote-box"> <p id="text">{quote.text}</p> <h2 id="author">{quote.author}</h2> <div class="click"> <button id="new-quote" class="btn" onClick={handleNewQuote} > New Quote </button> <a href="https://twitter.com/intent/tweet" id="tweet-quote" target="_blank" > Tweet </a> </div> </div>);
/*const getRandomIndex = (max) => Math.round(Math.random() * ((quoteData.length-1) - 0) + 0);*/
const getRandomIndex = (max) => Math.floor(Math.random() * (quoteData.length));
const App = () => { const [quote, setQuote] = React.useState(quoteData[getRandomIndex()])
const handleNewQuote = () => { setQuote(quoteData[getRandomIndex()]) } document.body.style.backgroundColor = backgroundColors[Math.floor(Math.random()*backgroundColors.length)];
return ( <div class="wrapper"> <QuoteBox handleNewQuote={handleNewQuote} quote={quote} /> </div> )}
ReactDOM.render(<App />, document.querySelector("#app"));
* { margin: 0; padding: 0; box-sizing:border-box;}
h2 { font-size: 19px; margin: 25px, 0; color: whitesmoke;}
p { margin: 0; font-size: 16px; color:whitesmoke;}
body { display: flex; width: 100vw; height: 100vh; justify-content: center; align-items: center; margin:auto; }
#quote-box { width: 340px; padding: 40px; border-radius: 10px; display:block; background:black; margin:auto;}
.click { display: flex; align-items: center;}
.wrapper .btn,#tweet-quote{ background:darkblue; display: flex; border-radius: 7px; padding: 7px 11px; cursor: pointer; font-size: 10px; color: whitesmoke; align-items:center; margin:25px 25px 25px; margin-bottom:-10px; margin-left:-7px; justify-content:center; }
#tweet-quote { margin-left: 7px; padding: 9px 15px; text-decoration: none; border:white; border-radius: 7px; }
Comments
Post a Comment