Variables in Python: Python For Beginners
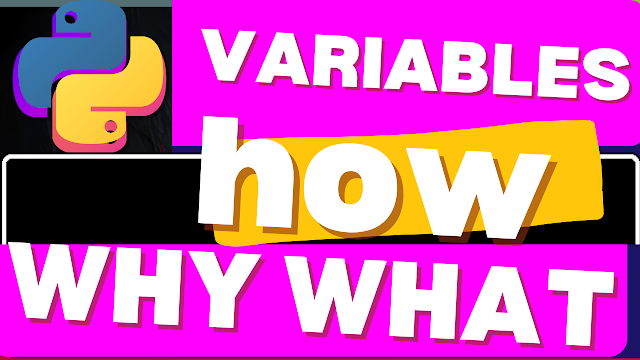
This tutorial will teach you how to build a simple dropdown menu in HTML and CSS for your website.
Building a simple navigation menu by using HTML and CSS will be an awesome practice for you to learn the basics of HTML and CSS and I will guide you on the basics of the code syntaxes used in this tutorial while building this menu.
In this navigation menu, we will be creating the menu for a fashion website, where we will have links to go to the Home page, and also links to take you to Bag, Shoe and Makeup pages. The idea of a menu in any website is for the user to navigate to different pages of the website or blog by clicking on the different tab links. So, we have the tabs for Home, Bag, Shoe and Makeup.
When there are several categories of products and each product having more than one sub-category, then, we need the feel to create a drop-down menu, whereby we can make the navigation much more simpler and less crowded. If you click on the category, you are shown all the sub-categories below the category tab, and it enables you to click on whichever sub-category that you want to click on, to bring you to that product in that range available.
Now we need to do the HTML code for this menu. Let's look at the code for the HTML below:
<!-dropdownmenu tutorial ->
<ul id='dropdownmenu'>
<li class="active"><a href='#'>HOME</a></li>
<li class="sub"><a href='#'>BAGS</a>
<ul>
<li><a href='#'>SLING</a></li>
<li><a href='#'>TOTE</a></li>
<li><a href='#'>CLUTCH</a></li>
</ul>
</li>
<li><a href='#'>SHOES</a>
<ul>
<li><a href='#'>HEELS</a></li>
<li><a href='#'>SPORTS</a></li>
<li><a href='#'>BOOTS</a></li>
</ul>
</li>
<li><a href='#'>MAKEUP</a>
<ul>
<li><a href='#'>LIPSTICK</a></li>
<li><a href='#'>EYELINER</a></li>
<li><a href='#'>MASCARA</a></li>
</ul>
</li>
</ul>
<!-end dropdownmenu->
We have given the id of "dropdownmenu" to the <ul> tag as this list is about a drop down menu. The 'active' class is added to a page in the menu in order to link to the page on which the user is currently active. In this case, we add the 'active' class to the home page in the menu. In between the list tags, we have the anchor tag, <a> and if you remember correctly, every anchor tag has a href attribute that helps to link to the destination page .In this case, the href attribute is given the value of '#' as we are doing this example menu for learning how to do and not pointing to any particular site. Had you wanted to link to another site or page within the same site or different site, then you need to write the complete url of the page or site inside of the # sign. In between the open and closed list tags, <li> and </li> tags, we write the name of the page that we want to show to the user, in this case, HOME. So, whatever you write in between the list tags would be visible to the user , but the list tags by itself would not be visible to the user. You can test it out in your code editor, to understand more clearly.
In whichever order, you want your website menu to appear, you need to list out in the same order. Here, we want to list the BAGS, then, SHOES and then MAKEUP , so, we will be listing out in the same order for the code.
Under the BAGS menu, we want to show three items, which are sling, tote, clutch respectively, so we list them in the same order. But, remember to write the <ul> tag for this sub list first before proceeding to write the <li> tags for individual items under the Bags list.
Hence, in order to avoid confusion in future, whenever you are making such drop down menu lists, just keep this formula in mind that for creating every list, remember the below formula or structure:
Hence, in the above scenario considering only one category X that has four items X1 to X4, it can be listed as shown above with the opening list tag of the X only closing after the closing of the <ul> tag of all the four items.
This is the structure of the tags that we are applying to the items in our website, namely, Bags, Shoes and Makeup. In the above example that I cited with X, since I am only considering one category, X, the </li> closed list tag happens after the closed </ul> tag. However, if we have more than one category, as in the case of our website, then, the closed </ul> tag will be written in the end after completing the set of code for individual category lists.
Let me cite with the above structural formula when there are categories X,Y and Z:
As you can note above, the code set for the three categories, with dropdown menu is the same for all the 3 sets . You can replace the X,Y and Z categories with the categories and their sub categories with what are there for your product sub categories. This is the code set I have followed for my website nav bar for the fashion website with categories, shoes, bags and makeup categories. Under Shoes category, we have heels, boots and sports and under Bags category, we have sling, tote and clutch and under makeup category, we have lipstick, eyeliner and mascara.
#dropdownmenu{
margin:34px 10px 10px -25px;
padding:10px 10px 10px 10px;
width: 3000px;
text-decoration :none;
l ist-style:none;
}
#dropdownmenu ul{
list-style:none;
height:20px;
margin:10px;
padding:10px;
/*margin:10px;*/
/*padding:10px;*/
}
#dropdownmenu li{
float:left;
padding:6px;
margin-left:-3px;
}
#dropdownmenu li a{
text-decoration: none;
}
#dropdownmenu li > a:hover, #dropdownmenu ul li:hover{
background-color: blueviolet;
}
#dropdownmenu li ul {
display:none;
/*height:auto;*/
padding:0px;
margin:0px;
position:absolute ;
height:auto;
width:100px;
}
#dropdownmenu li:hover ul{
display:block;
}
#dropdownmenu li ul a{
display:block;
height:auto
padding:auto;
height :auto;
text-align:left;
color:whitesmoke;
background:darkcyan;
}
#dropdownmenu a {
color:whitesmoke;
background:deeppink;
padding:4px;
height:auto;
}
In the above the menu with id of dropdownmenu has a list-style-type set to a value of none and text-decoration value set to none. The list-style-type is actually an item market for the list, and it is totally upto you if you like to have an item market or have no market at all. I would suggest that you experiment with different values such as decimal, circle, square, disc etc and see how the list is marked in your final outcome.
For the text-decoration property, you could have various styles such as colors, lines, line-throughs, thickness, etc, In the above website navbar example that I am teaching, I have set the value of text-decoration to none.
For the list items, the float value is set to left that means the alignment is from left to right direction.
We can add hover properties such that the color of the navbar changes when we click on the individual nav links. I would suggest you to experiment with different colors and not just limit yourself to the color code that I have provided in the above code for the hover property.
Since we want the navbar to take up the whole width of the page, we give the value of block to the display property to the navbar ul. The CSS used here is of a very basic nature, so, with the simple explanation of the essentials above, I hope, you have understood the styling of this dropdown menu quite easily. If there is something that you do not follow, you are welcome to drop your comments below in the comment section, and I will be only too glad to be of help to solve your doubts.
The building of this beautiful yet simple dropdown menu for your website was done with minimum code in HTML and CSS. It becomes more colorful due to the hover properties that we have used, as you can see from the outcome in your code editor. I suggest that you use either codepen or JSitor if you are new to coding so that you find it easier to deal with the code editor's simple editor modes.
Moreover, you can sign up for both the above code editors with a free account so , no need to spend a fortune when you are in the beginning of your programming learning journey,
You can modify the css code that I have used or you can use the same code, but, I suggest that you give it a tweak by modifying the background colors that I have used and also the padding, margin, width, etc, so that you get to experiment with various design elements while you are coding and this experience would give you more confidence in your coding path.
Comments
Post a Comment